3. Recursion¶
Don’t think too hard! That’s one of the central themes of this chapter. It’s not often that you tell computer programmers not to think too hard, but this is one time when it is appropriate. You need to read this chapter if you have not written recursive functions before. Most computer science students start by learning to program in a style called imperative programming. This simply means that you are likely used to thinking about creating variables, storing values, and updating those values as a program proceeds. In this chapter you are going to begin learning a different style of programming called functional programming. When you program in the functional style, you think much more about the definition of what you are programming than how you are going to program it. Some say that writing recursive functions is a declarative approach rather than an imperative approach. You’ll start to learn what that means for you very soon. When you start to get good at writing recursive functions you’ll be surprised how easy it can be!
3.1. Scope¶
You can download the program
by clicking here.
1import math
2
3
4PI = math.pi
5
6def area(radius):
7 global z
8 z = 6
9 theArea = PI * radius ** 2
10
11 return theArea
12
13
14def main():
15 global z
16
17 historyOfPrompts = []
18 historyOfOutput = []
19
20 def getInput(prompt):
21 x = input(prompt)
22 historyOfPrompts.append(prompt)
23
24 return x
25
26 def showOutput(val):
27 historyOfOutput.append(val)
28 print(val)
29
30 rString = getInput("Please enter the radius of a circle: ")
31
32 r = float(rString)
33
34 val = area(r)
35 print(z)
36 showOutput("The area of the circle is " + str(val))
37
38if __name__ == "__main__":
39 main()
3.2. Recursive Spiral¶
You can download the program
by clicking here.
1import turtle
2
3def drawSpiral(t, length, color, colorBase):
4 #color is a 24 bit value that is changing a bit
5 #each time for a nice color effect
6 if length == 0:
7 return
8
9 # add 2^10 to the old color modulo 2^24
10 # the modulo 2^24 prevents the color from
11 # getting too big.
12 newcolor = (int(color[1:],16) + 2**10)%(2**24)
13
14 # find the color base integer value
15 base = int(colorBase[1:],16)
16
17 # now if the new color is less than the base
18 # add the base module 2^24.
19 if newcolor < base:
20 newcolor = (newcolor + base)%(2**24)
21
22 # let newcolor be the hex string after conversion.
23 newcolor = hex(newcolor)[2:]
24
25 # add a pound sign and zeroes to the front so it
26 # is 6 characters long plus the pound sign for a
27 # proper color string.
28 newcolor = "#"+("0"*(6-len(newcolor)))+newcolor
29
30 t.color(newcolor)
31 t.forward(length)
32 t.left(90)
33
34 drawSpiral(t, length-1, newcolor, colorBase)
35
36def main():
37 t = turtle.Turtle()
38 screen = t.getscreen()
39 t.speed(100)
40 t.penup()
41 t.goto(-100,-100)
42 t.pendown()
43
44 drawSpiral(t, 200, "#000000", "#ff00ff")
45
46 screen.exitonclick()
47
48
49if __name__ == "__main__":
50 main()
51
3.3. Sunflower Program¶
You can download the program
by clicking here.
This program is not included in the text, but is a good program to look at when starting chapter 3. It illustrates the golden rule and the fibonacci sequence. Computing fibonacci numbers both recursively and iteratively can lead to a good discussion of computational complexity as well as recursion. You can write a program to time computing fibonacci numbers both recursively and iteratively. The recursive version will not handle numbers bigger than 20 or so. The iterative version can go very high. It is interesting to look at the graph of these two methods of computing fibonacci numbers as a contrast in what is efficient and what is not.
1'''
2 Author: Kent D. Lee
3 Date: 9/26/2014
4 Copyright (c) 2014
5 Free for educational use. Others may use with permission.
6
7 Source:
8
9 I used http://fractalfoundation.org/OFC/OFC-11-3.html as a source for this
10 information.
11
12 Description:
13
14 This program draws sunflower seeds in the pattern of a funflower. The ration of
15 consecutive fibonacci numbers in the sequence approach the golden ratio as the
16 sequence grows. In the limit, the ratio of two consecutive fibonacci numbers is
17 the golden ratio.
18
19 In the sunflower fibonacci numbers can be observed by counting the number of seeds
20 in the spiral arms. Count the number of seeds in a left spiral arm and a right spiral
21 arm. You'll see that they are two fibonacci numbers.
22
23 You may have to make the radius size of the seeds constant to count the seeds. It won't
24 look as pretty, but will be easier to count. You may also need to increase the forward
25 to separate the seeds.
26'''
27
28import turtle
29import math
30
31
32class Circle:
33 def __init__(self,radius, width=1,color="white",outline="black"):
34 self.radius = radius
35 self.width = width
36 self.color = color
37 self.outline = outline
38
39 def draw(self,turtle):
40 centerX = turtle.xcor()
41 centerY = turtle.ycor()
42 turtle.penup()
43 turtle.goto(centerX+self.radius,centerY)
44 turtle.pendown()
45 turtle.width(self.width)
46 turtle.pencolor(self.outline)
47 turtle.fillcolor(self.color)
48 turtle.begin_fill()
49 for i in range(361):
50 newX = self.radius * math.cos((i/180.0) * math.pi) + centerX
51 newY = self.radius * math.sin((i/180.0) * math.pi) + centerY
52 turtle.goto(newX,newY)
53
54 turtle.end_fill()
55 turtle.penup()
56 turtle.goto(centerX, centerY)
57 turtle.pendown()
58
59def main():
60
61 t = turtle.Turtle()
62 t.ht()
63 screen = t.getscreen()
64 screen.tracer(0)
65
66 for x in range(400):
67 c = Circle(x/16+4,width=2,color="yellow")
68 c.draw(t)
69 # This angle is chosen because it is approx.
70 # 360/1.61803399. The 1.61803399 is the approx.
71 # value of the golden angle
72 t.left(222.5)
73 t.penup()
74 t.forward(x*2 + 8)
75 screen.update()
76
77
78
79 screen.exitonclick()
80
81if __name__ == "__main__":
82 main()
3.4. Figures from Text¶
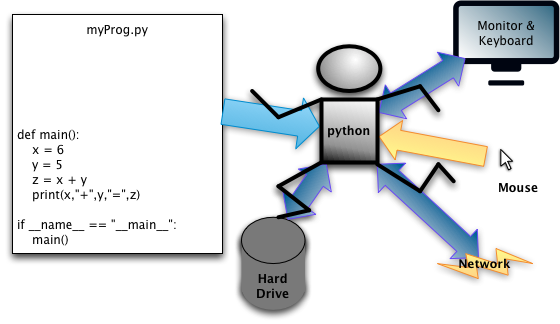
Fig. 1: The Python Interpreter¶
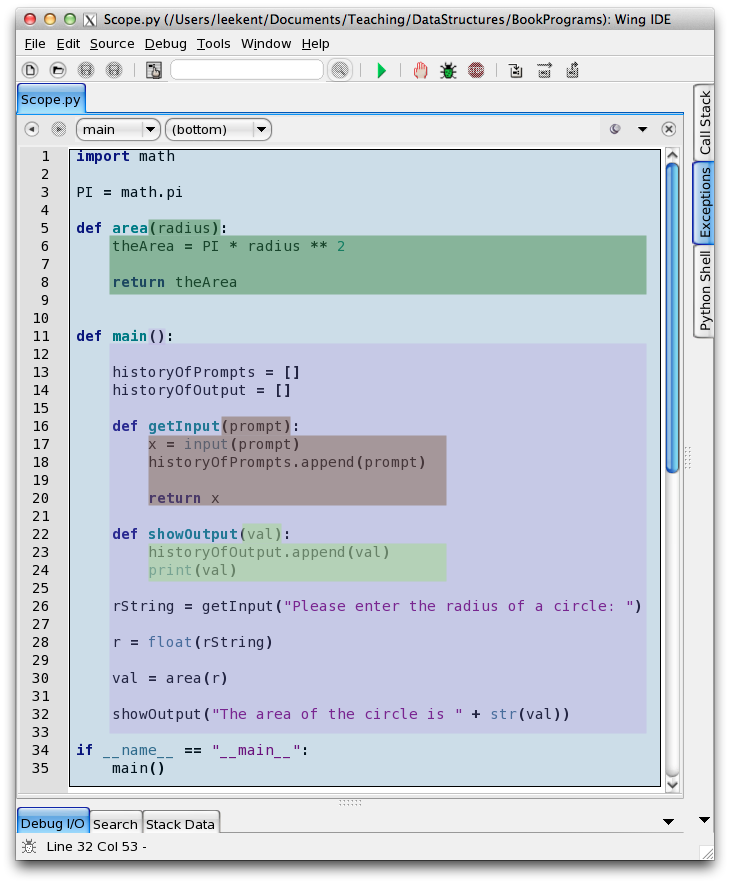
Fig. 2: Scopes within a Simple Program¶
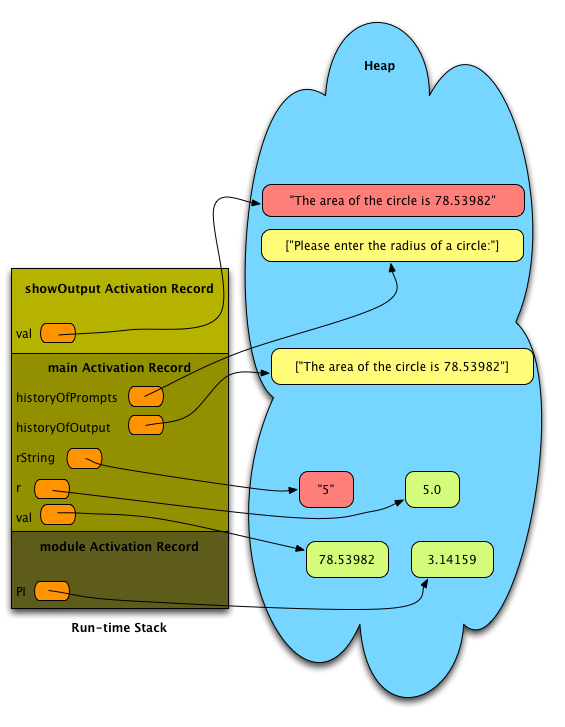
Fig. 3: The Run-time Stack and the Heap¶
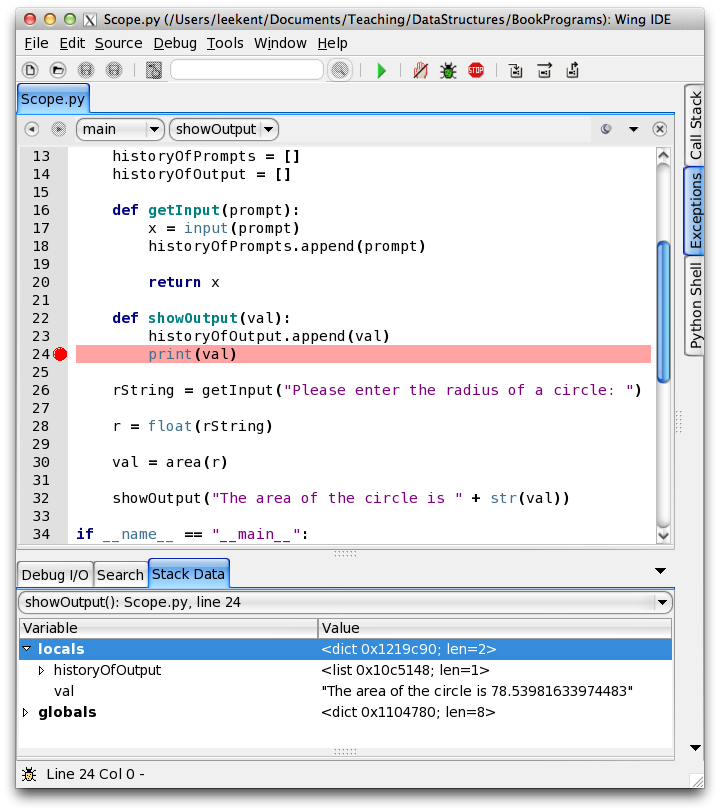
Fig. 4: The Wing IDE Showing the Run-time Stack¶
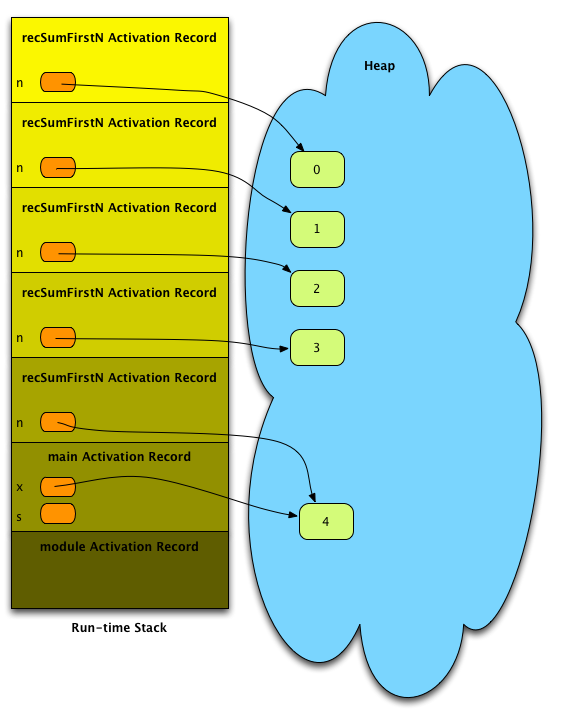
Fig. 5: The Run-time Stack of a Recursive Function Call¶
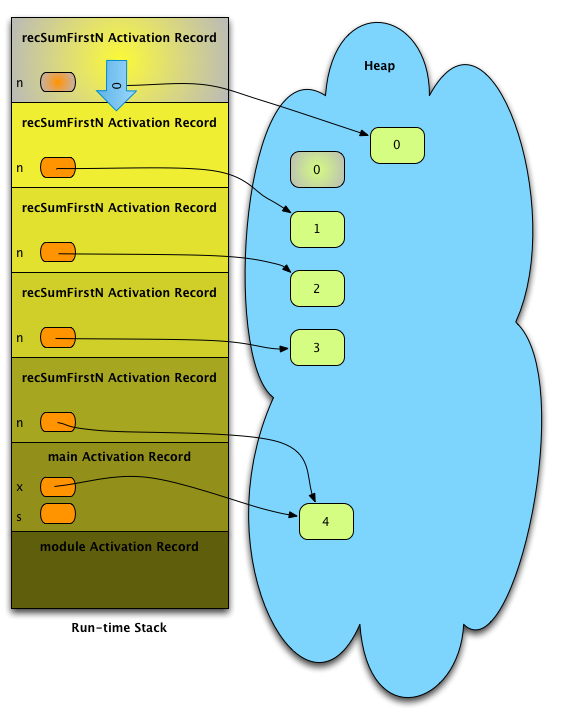
Fig. 6: The First Return from recSumFirstN¶
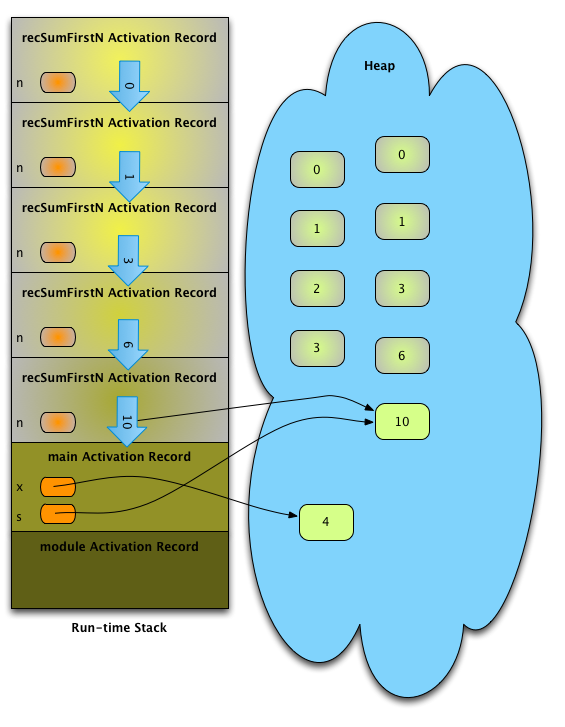
Fig. 7: The Last Return from recSumFirstN¶
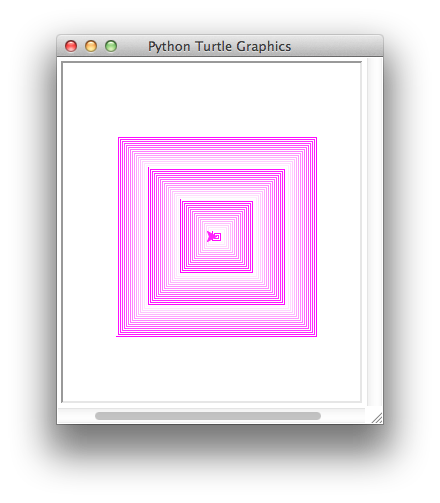
Fig. 8: A Spiral Image¶
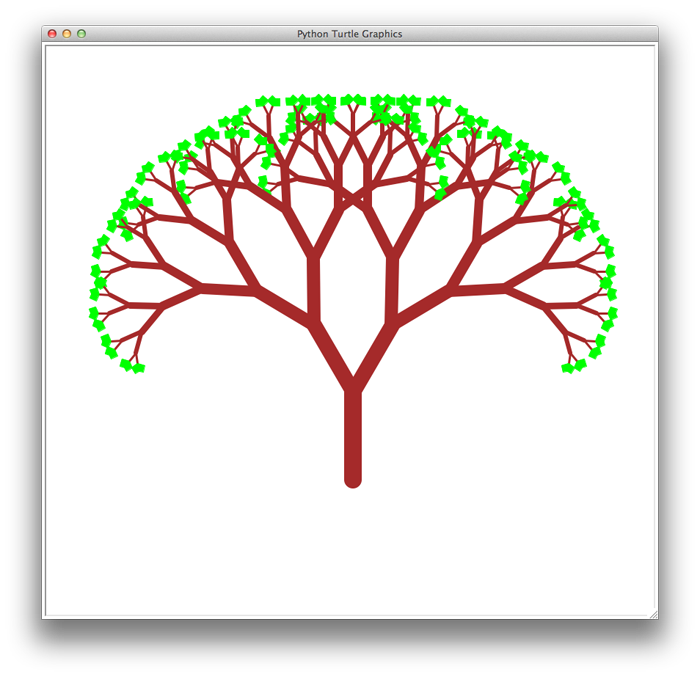
Fig. 9: A Tree¶