Welcome!¶
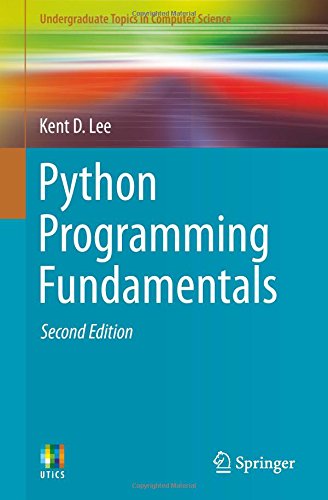
Welcome to Python Programming Fundamentals Second Edition by Kent D. Lee. This text, available from Springer, is an introductory computer programming text. The text is oriented towards students who have not taken any other programming course previously. However, it can be used by students with some programming skills in another language. If you would like to begin learning Python programming on your own, you can begin by installing Python and Wing IDE 101 on your computer. Then buy my book and begin working through the many practice exercises and examples in the text. The links to the two YouTube videos below show you how you can install Python and Wing IDE 101 on your computer.
I hope you enjoy reading the text and learning to program a computer using Python! It’s creative, fun, and the skills you will learn could provide you with access to a very fullfilling and rewarding career in Computer Science! Give it a try!
Errata and Suggestions¶
I hope both professors and students enjoy using the text! I hope to get constructive feedback from folks so if you find an error or have a suggestion don’t hesitate to email me. My email is kentdlee at luther.edu. Please contact me via email if you have errata or suggestions and I will post them here.
On page 22. The sentence containing “Dividing 83/2 yields 41.5 if it is written 81/2” should be “… if it is written 83/2”.
Instructor Support Materials¶
If you are an instructor using or considering using the text to teach a course and would like lecture slides, solutions to exercises, and answers to review questions I would be happy to provide them. Detailed lecture slides are available with all examples and practice problems from the text as well as solutions to all the exercises and review questions from the text. Please email me and provide me with information about where you are teaching and a web site where I can verify that you are a teacher at your institution and I will direct you to these support materials.
Support Files for Individual Chapters¶
The following sections contain support files, instructional videos, and additional information for individual chapters. The support files may be downloaded by students or faculty to complete projects from each of the chapters below.
The sections below also contain videos for the various topics presented in the text. These videos help to reinforce the topics discussed in the text. I would recommend that you watch the video and then read the section of the text that goes along with the video for a more detailed explanation of the concepts in that section.
In addition, there are practice exercises in each section of the text (with answers at the end of the chapter) that you can use to test your understanding material. Use these exercises to motivate your reading of the text.
Chapter 1¶
The first chapter is about 30 pages long and contains a variety of learning objectives. This is perhaps the most broadly focused of the chapters in the text. Its goal is to give you some basis for talking about computer programming and to give you a gentle introduction to the process of writing programs. Specifically, the learning objectives of the first chapter are outlined below.
Section 1.3 - In this section of the text you use the Wing IDE to write a simple program. You learn the steps that you must take to open the Wing IDE, enter a program, and run it.
Section 1.4 - In this section you learn a little about the architecture of a computer and some general terms to use when talking about computer programs.
Sections 1.5 to 1.7 Part 1 - Covers conversion to binary.
Sections 1.5 to 1.7 Part 2 - Signed and unsigned binary number representation is discussed.
Sections 1.5 to 1.7 Part 3 - A first algorithm is presented and discussed.
Sections 1.5 to 1.8 Part 1 - In these sections of the text, you learn about the importance of types in our programs and ASCII representation of characters.
Sections 1.5 to 1.8 Part 2 - In this video you learn to use an assignment statement in Python to store a value in RAM and retrieve it later.
Sections 1.9 and 1.17 - In section 1.9 and 1.17 you learn what a syntax error is, how to recognize it in your program, and how to fix it.
Sections 1.10 to 1.14 - In this part of the text you learn about references, types, and type conversion.
Sections 1.15 & 1.16 - Here you practice with strings and getting input from the user and printing output.
Chapter 2¶
In chapter 2 you learn how to make decisions in your programs using if statements. An if statements alters the sequence of executed statements depending on a condition. You also learn about the guess and check pattern of computation. This pattern is important to learn and memorize because you use it over and over in programs you write. Learning objectives for this chapter are outlined below.
Sections 2.1 & 2.2 - Here you learn about the guess and check pattern of computation. You can watch this video that goes along with these chapters.
Sections 2.3, 2.4, & 2.5 - In these sections you learn about choosing from a list of alternatives, Boolean values, and short-circuit logic. The video below helps to further explain these concepts.
Section 2.6 - In this section we learn that floating point numbers are only approximations of real numbers and therefore we must take some care when comparing floats for equality. It is likely that two floats will be close to the same value, but not exactly the same value, even when we would like them to be equal. The video below helps to illustrate this problem and the solution to it.
Chapter 2 Support Files¶
In exercise 2 of chapter 3 the problem asks you to write a menu driven address book program. You can use this text file as the data file for your address book or you can create one yourself. Just be sure to use the same format as is presented in the book for your records within the file.
Chapter 3¶
In chapter 3 you learn how to repeatedly execute code in programs so you can process repetitive data. This is where computers really are important, since people don’t like to do the same thing over and over again. Computers are good at doing the same thing over and over. Again, there are video lectures below that complement the text.
Sections 3.1 & 3.2 - You should read the introduction to chapter 3 as well. In these two sections you learn about sequences and how to iterate over them.
Section 3.3 - In this section you learn about nested for loops. Nested for loops are when you have one loop inside another loop.
Section 3.4 - In this section of the text you learn how to use the guess and check pattern when a list is involved.
Sections 3.5 & 3.6 - In these sections of the text you learn about the accumulator pattern and how to use it to count or add together a list of numbers.
Chapter 4¶
In chapter 4 you learn about objects and how to create objects and call methods on them. The chapter uses turtle graphics and XML parsing as two examples where many objects are created and methods are called.
Sections 4.1 to 4.3 - The introduction and these sections of the text show you how to use modules in Python and how to create some objects. This video complements these sections of the text.
Plotting Data - In this video you learn how to use turtle graphics to plot data that you read from a file. In this video I read the file called djia-100.txt. This file is provided here for you to download if you want to try out my example.
Section 4.8 - In this section of the text you learn about dictionaries in Python. It shows you how to create a dictionary, put a key/value pair in dictionary, and how to lookup a key in a dictionary to get its value. The video below demonstrates these concepts as well.
Sections 4.6 to 4.9 - In these sections of the text you learn how to read and parse through the information in an XML file. XML documents are used in many different applications these days and understanding what an XML document is and how to read it in a program is a valuable skill. The video below helps to illustrate these ideas. This video use an XML file called kml.kml as an example. That file can be downloaded here if you want to try my example.
Section 4.7 - In this section of the text I show you how to get at the attributes in an XML element. The attributes are recorded as a dictionary so you’ll want to know what a dictionary is first (see section 4.8 and its video above). In the video that goes along with this section I read a couple of XML files called biking3-15-2012.tcx and workouts.tcx. You can download these files here if you want to try my examples, but these files are large and a little unwieldy. You might start with some smaller XML files first (see the exercises at the end of the chapter in the text).
Support Files¶
In chapter 4 there are several programming problems that must read data from a file. In a few cases, some code is provided to get you started. Each exercise’s extra files are provided below.
Exercises 2, 3, and 4 require you to read an XML file containing a picture. The XML file can be generated by using this drawing program, written in Python. You can download this program and run it with Python 3 to be able to draw a picture and save it to an XML file.
The XML file for the picture in the text (Fig. 4.4 page 99) can be found here as flowerandbg.xml.
- Exercises 5 and 6 use mileage and fuel data from a 2007 Toyota 4Runner, a 2007 Nissan Versa, and a 2007 Suzuki S40 motorcycle. These two programs require you to read one of these data files below. This data was exported from a program called Gas Cubby that is available as an iPhone/iPod Touch App.
Toyota4Runner.csv - Data for gas mileage for a 2007 Toyota 4Runner.
NissanVersa.csv - Data for gas mileage for a 2009 Nissan Versa.
SuzukiS40.csv - Data for gas mileage for a 2007 Suzuki S40 Motorcycle.
Chapter 5¶
Chapter 5 introduces you to defining and calling functions of your own. Functions offer us a means of abstracting away details and concentrating on what a function does instead of how it does it.
Defining and calling functions is discussed in this video and in sections 5.1 and 5.2 of the text.
Functions and Scope are described in section 5.3 of the text and this video lecture.
The Run-time Stack is described in this video lecture and section 5.4 of the text.
Bottom-up Design is described in section 5.8 of the text and this video lecture.
The Main Function is described at the end of chapter 5 and in this video lecture.
Chapter 6¶
Chapter 6 introduces Tkinter programming through an example Reminder application. Documentation for the Tkinter API can be found here. You can download the Reminder.py code from the example in that chapter here. Exercise 4 requires you to create an XML file to hold the reminder notes when the program is terminated and for the program to read when the application is started. You can use the format found below for your XML file. Name the file “Reminders.xml” and place the xml file in the same directory or folder as your program. You can begin this exercise by downloading the Reminder.py program from the link above and then modifying the code to read from an XML file using the minidom parser described in chapter 4. You can write to a file using Python’s file write method like the code in Reminder.py already does. Of course, you need to modify the code to write the file in this XML format so it can be read by the minidom parser.
One thing to be aware of: When using double quotes (i.e. “) in strings, you can you single quotes (i.e. ‘) to delimit those strings. Python lets you use either double or single quotes to delimit strings and this is one instance where using single quotes will come in handy.
One other thing to be aware of: Sometimes exception handling code (i.e. try except statements) get in the way of debugging your code. You may want to remove (or just comment out) the try except statements in the Reminders.py program before making modifications to it so you can see what errors occur when you make the modifications for this assignment. You should put the try except statements back in once you have debugged your code.
<?xml version="1.0" encoding="UTF-8" standalone="no" ?>
<Reminders x="121" y="76">
<Note x="205" y="374">
Take out garbage.
</Note>
<Note x="132" y="602">
Pick up mower blades.
</Note>
</Reminders>
There is one video lecture for chapter 6 that goes along with the material in all of chapter 6 and teaches you about tkinter programming.
Support Files¶
You can find the Reminder Application here as it is described in chapter 6 of the text.
Chapter 7¶
In chapter 7 there are two sample programs that are developed to demonstrate object-oriented programming and inheritance using Turtle graphics.
There are two video lectures that go along with this chapter.
Object-Oriented Programming and creating and using classes in described in this video.
Inheritance is described in this lecture and the reuse of code through inheritance.
Support Files¶
The first sample program is a drawing application. The source code for the drawing program can be downloaded here.
The second example is a bouncing ball program and that code can be downloaded here. The bouncing ball program needs the soccerball.gif file to run. You might also like to make kitties bounce around, so kitty.gif is also provided.
Chapter 7 includes an exercise on developing an asteroids application. There are five lessons that will take you through developing this application in some earlier materials called Sampling CS The five lessons below can be followed to implement the basic asteroids application. As part of the assignment in the text, you are to implement one more level to the application when level 1 is completed. The five lessons for the Asteroids game start with lesson 11 and links to them can be found at this location.
In addition to the exercises at the end of chapter 7 I have had students implement a minesweeper game in the past. The Game Development with Python website contains lessons for developing games in Python including the minesweeper game (see lessons 14-18). These lessons will help guide students through building their own minesweeper application.